Step-by-Step Process of Implementing Stemming and Lemmatization in Python?
Naveen
- 0
Install the Natural Language Toolkit (NLTK) library. This library provides a range of tools for natural language processing, including stemming and lemmatization algorithms. You can install it using pip install nltk.
Import the necessary functions from the NLTK library. For example, to use the Porter stemmer, you would use the following import statement: from nltk.stem.porter import PorterStemmer. To use the WordNetLemmatizer, you would use the following import statement: from nltk.stem import WordNetLemmatizer.
Tokenize the text that you want to stem or lemmatize. This involves splitting the text into individual words or tokens. You can use the nltk.tokenize module to do this, or you can write your own tokenization function.
Create an instance of the stemmer or lemmatizer class. For example, to create an instance of the Porter stemmer, you would use the following code: stemmer = PorterStemmer(). To create an instance of the WordNet lemmatizer, you would use the following code: lemmatizer = WordNetLemmatizer().
Iterate over the tokens and apply the stemmer or lemmatizer to each token. For example, to stem a token using the Porter stemmer, you would use the following code: stemmed_token = stemmer.stem(token). To lemmatize a token using the WordNetLemmatizer, you would use the following code: lemmatized_token = lemmatizer.lemmatize(token).
Optionally, you can join the stemmed or lemmatized tokens back into a single string using ‘ ‘.join() function.
Here is an example of how to implement stemming in Python using the Porter stemmer from the NLTK library:

To stem a single word, you can simply pass the word to the stem method of the stemmer object. For example, to stem the word “running”, you would use the following code: stemmed_word = stemmer.stem(“running”).
To stem a list of words, you can use a list comprehension or a loop to iterate over the words and stem each one individually. You can then store the stemmed words in a list or use them directly in your code.
It is important to note that stemming can affect the meaning of the words, as it involves reducing them to their base form. This can be useful for certain tasks such as text classification, but it can also be problematic for tasks that require a more nuanced understanding of language. It is important to consider the impact of stemming on the performance of your model.
Lemmatization
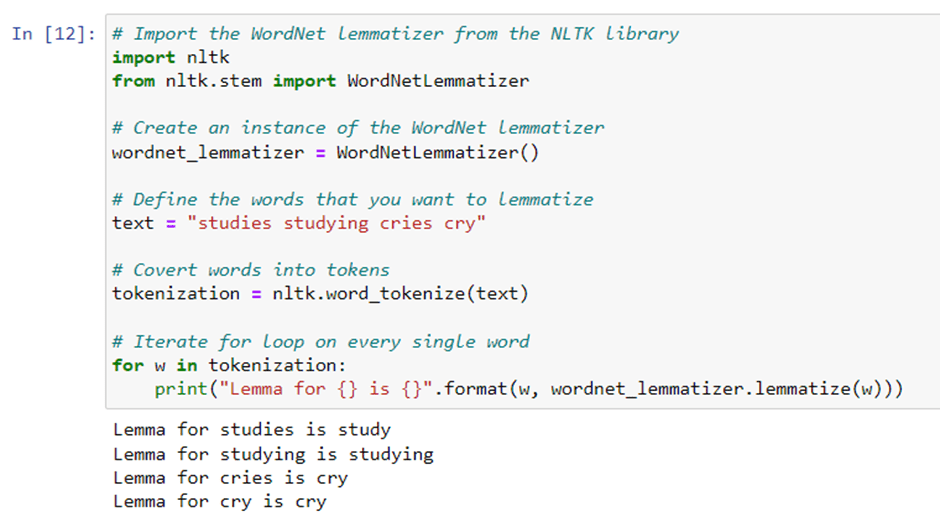
To lemmatize a single word, you can simply pass the word to the lemmatize method of the lemmatizer object. For example, to lemmatize the word “running”, you would use the following code: lemmatized_word = lemmatizer.lemmatize(“running”).
To lemmatize a list of words, you can use a list comprehension or a loop to iterate over the words and lemmatize each one individually. You can then store the lemmatized words in a list or use them directly in your code.
It is important to note that lemmatization takes into account the part of speech and inflection of the words, and therefore may produce different results than stemming. Lemmatization can be more linguistically accurate but may be more computationally expensive than stemming. It is important to consider the trade-offs between lemmatization and stemming for your specific task.