What is List comprehension and how to use it?
Naveen
- 0
List comprehension is a concise way to create a list using a single line of code. It consists of square brackets containing an expression followed by a for clause, then zero or more for or if clauses. The expressions can be anything, meaning you can put in all kinds of objects in lists.
Example 1: Create a list of the squares of the numbers 0 through 9:
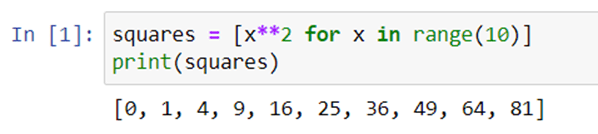
You can also include an if clause in your list comprehension to filter the list. For example, the following list comprehension creates a list of the squares of the even numbers between 0 and 9:

List comprehension is often used to process data in a list and create a new list. For example, suppose you have a list of strings and you want to create a new list that contains only the strings that are uppercase. You can use the following list comprehension to do this:

Example 2: Create a list of the first 10 positive integers and their squares:

Example 3: Create a list of the first 10 positive integers and their squares, but only include the integers and their squares if the integer is odd:

Example 4: Create a list of the first 10 positive integers and their squares, but only include the integers and their squares if the integer is even and the square is greater than 25:

Example 5: Create a list of the first 10 positive integers and their squares, but only include the integers and their squares if the integer is odd or the square is greater than 25:

Why do we use List Comprehension?
List comprehension is a concise way to create a list using a single line of code. It is often used as a more efficient alternative to a for loop, especially when you want to create a new list based on the values of an existing list.
List comprehension is particularly useful when you want to perform some operation on each element of a list and create a new list with the results. For example, suppose you have a list of strings and you want to create a new list that contains only the strings that are uppercase. You could do this using a for loop as follows:
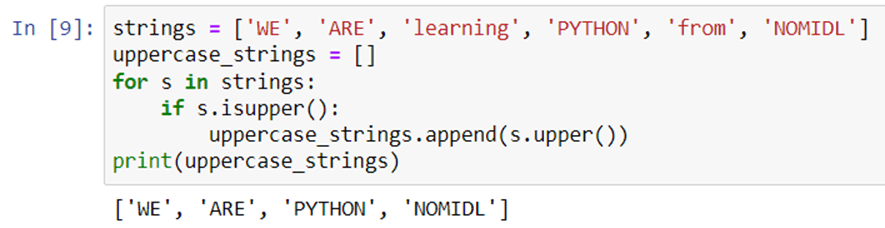
However, this can be done more concisely and efficiently using list comprehension as follows:

List comprehension is also useful for creating lists of complex data structures, such as lists of lists or lists of dictionaries.
In summary, list comprehension is a useful tool in Python for creating lists efficiently and concisely, and is particularly useful when you want to perform some operation on each element of a list and create a new list with the results.
What are the Advantages of using List Comprehension?
There are several advantages to using list comprehension in Python:
1 – Concise syntax: List comprehension allows you to create a list using a single line of code, which can make your code more readable and easier to understand.
2 – Faster execution: List comprehension is often faster than using a for loop to create a list. This is because list comprehension involves less code and can take advantage of optimized code paths in the Python interpreter.
3 – More readable code: List comprehension can make your code more readable because it allows you to express your intentions more clearly. For example, you can use list comprehension to create a list of the squares of the first 10 positive integers as follows:

This is more readable than using a for loop to accomplish the same task:

4 – More efficient code: List comprehension can be more efficient than using a for loop because it involves less code and can take advantage of optimized code paths in the Python interpreter.
In summary, list comprehension offers concise syntax, faster execution, more readable code, and improved efficiency, making it a useful tool for creating lists in Python.
What is the difference between List and List comprehension?
The main difference between a list and list comprehension in Python is how they are constructed. A list is created using square brackets and a list of elements, separated by commas. For example:

List comprehension, on the other hand, is a concise way to create a list using a single line of code. It consists of square brackets containing an expression followed by a for clause, then zero or more for or if clauses. For example:

This list comprehension creates a list of the squares of the numbers 1 through 5.
Another difference is that list comprehension is often more efficient and concise than using a for loop to create a list. List comprehension allows you to express your intentions more clearly and can take advantage of optimized code paths in the Python interpreter, resulting in faster execution and more efficient code.
In summary, the main difference between a list and list comprehension in Python is that a list is created using a list of elements, while list comprehension is a concise way to create a list using a single line of code that includes an expression and one or more for or if clauses. List comprehension is often more efficient and concise than using a for loop to create a list.