Efficient Data Manipulation with Apply() Function in Pandas
Naveen
- 0
If you’re a data enthusiast like me, you’ve probably dabbled in the world of Python and Pandas, the go-to library for data manipulation and analysis. Now, imagine you have a massive dataset with thousands of rows, and you want to perform some custom operations on it. Well, don’t fret! Pandas has your back, and it comes bearing a powerful tool called the apply()
function. Let’s dive into this magical world of efficient data manipulation and uncover the secrets of the apply()
function.
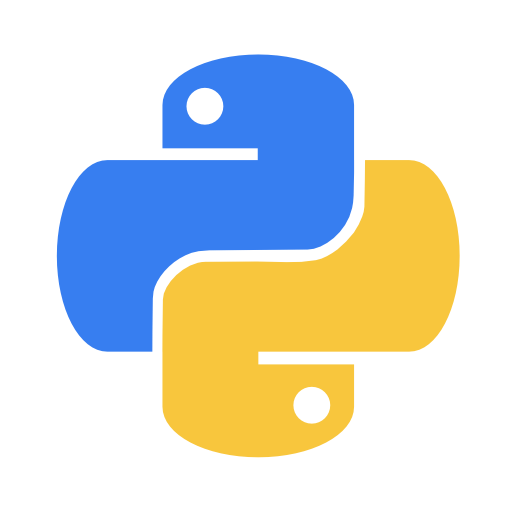
Apply()
You’re running a small online business, and you have a Pandas DataFrame containing all your customer orders. Each row in the DataFrame represents an order, and you want to calculate the total price for each order, including taxes and discounts.
Now, before the apply()
function entered the stage, you’d have to loop through each row of the DataFrame, perform the calculations, and then store the results. Not only would this be time-consuming, but it would also be a coding nightmare, with potential pitfalls at every turn.
That’s where the apply()
function comes to save the day! It’s like having a magical wand that effortlessly performs operations on your data. The apply()
function allows you to apply any custom function to each row or column of your DataFrame, and it does it with lightning speed!
Let’s Break It Down: Basic Syntax
The basic syntax of the apply()
function looks like this
df['new_column'] = df['existing_column'].apply(your_custom_function)
See, it’s not rocket science! You select the column you want to work on, use the apply()
function, pass in your custom function, and voila! A new column with the results of your function is created.
Making Magic Happen with Real-Life Examples
Alright, let’s roll up our sleeves and unleash the true power of apply()
with some real-life examples.
Example 1: Calculating Total Order Price
Remember our online business scenario? Here’s how we can calculate the total order price using ‘apply()'
:
import pandas as pd # Sample DataFrame representing customer orders data = {'Order_ID': [101, 102, 103], 'Product_Price': [25.99, 14.50, 8.75], 'Tax_Percentage': [0.08, 0.1, 0.05], 'Discount_Percentage': [0.2, 0.1, 0.15]} df = pd.DataFrame(data) # Custom function to calculate total order price def calculate_total_price(row): product_price = row['Product_Price'] tax_percentage = row['Tax_Percentage'] discount_percentage = row['Discount_Percentage'] total_price = product_price + (product_price * tax_percentage) - (product_price * discount_percentage) return total_price # Applying the custom function using apply() df['Total_Price'] = df.apply(calculate_total_price, axis=1) print(df)

And just like that, we have a new column in our DataFrame called Total_Price
, containing the calculated total price for each order!
Example 2: String Manipulation
Let’s say you have a column with messy strings, and you want to clean them up. Here’s how apply()
can come to your help:
# Sample DataFrame with messy strings data = {'Names': ['JoHN', 'sARAh', 'mICHeLLe']} df = pd.DataFrame(data) # Custom function to clean up names def clean_name(name): return name.title() # Applying the custom function using apply() df['Clean_Names'] = df['Names'].apply(clean_name) print(df)
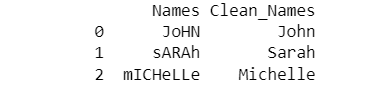
Now, our DataFrame has a new column called Clean_Names
with beautifully formatted names!
Also read: 10 Common Pandas Errors and How to Fix Them
The Hidden Efficiency of Apply()
Apart from its flexibility and ease of use, apply()
has an underlying efficiency that might surprise you. When you use apply()
, Pandas optimizes the process to run efficiently in the background, making it quite fast even on large datasets.
When to Use Apply() Wisely
While apply()
is a powerful tool, like all magic, it’s essential to use it wisely to get the most out of it.
1. Small, Frequent Tasks: Use apply()
for small, frequent tasks where its convenience outweighs any performance considerations. For larger tasks, there might be more efficient Pandas methods available.
2. Built-in Functions vs. Custom Functions: Before reaching for a custom function, check if Pandas already provides a built-in function that can achieve the same result. Built-in functions are often faster and more optimized.
3. Vectorized Operations: Pandas thrives on vectorized operations, where you apply functions to entire Series or DataFrames at once, rather than row by row. Whenever possible, try to leverage these vectorized operations for faster execution.
Conclusion
In the world of data manipulation with Pandas, the apply()
function is like a versatile wand that empowers you to perform custom operations with ease. From complex calculations to simple string manipulations, apply()
can handle it all, making your data manipulation tasks a breeze.
So, the next time you find yourself facing a daunting data challenge, remember to reach for the apply()
function.
If you found this article helpful and insightful, I would greatly appreciate your support. You can show your appreciation by clicking on the button below. Thank you for taking the time to read this article.
Popular Posts
- From Zero to Hero: The Ultimate PyTorch Tutorial for Machine Learning Enthusiasts
- Day 3: Deep Learning vs. Machine Learning: Key Differences Explained
- Retrieving Dictionary Keys and Values in Python
- Day 2: 14 Types of Neural Networks and their Applications