Retrieving Dictionary Keys and Values in Python
Naveen
- 0
Python is a versatile and powerful programming language that provides many ways to retrieve keys and values from dictionaries. Dictionaries are a fundamental data structure in Python, allowing you to store key-value pairs for efficient lookup and manipulation. In this article, we will see different methods to retrieve keys and values from dictionaries with code examples. So, let’s dive in!
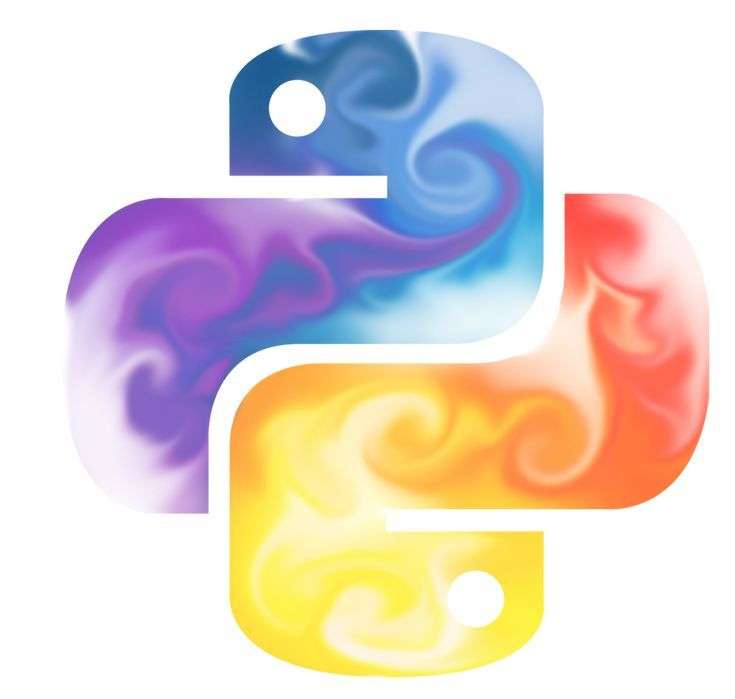
Introduction
Dictionaries in Python are unordered collections of key-value pairs enclosed in curly braces {}. They provide a fast and efficient way to store and retrieve data based on unique keys. To work effectively with dictionaries, it is important to understand how keys and values can be retrieved from them.
Accessing Keys and Values Using Loops
Python offers loop constructs such as for and while that allow us to iterate over dictionaries and retrieve their keys and values.
Retrieving Keys
All keys can be retrieved from the dictionary using a for loop using the keys() method. Let’s see an example:
my_dict = {'name': 'Ajay', 'age': 23, 'city': 'Mumbai'} for key in my_dict.keys(): print(key)
output
name age city
Retrieving Values
Similarly, we can also use a for loop with the value() method to retrieve all values from the dictionary. Let’s see an example:
my_dict = {'name': 'Ajay', 'age': 23, 'city': 'Mumbai'} for value in my_dict.values(): print(value)
output
Ajay 23 Mumbai
Accessing Keys and Values Using Dictionary Methods
Apart from loops, Python provides convenient dictionary methods to retrieve keys and values.
Retrieving Keys
The keys() method returns a view object that contains all the keys of a dictionary. We can convert this view object into a list if needed. Consider the following example:
my_dict = {'name': 'Sandesh', 'age': 24, 'city': 'New Delhi'} keys = list(my_dict.keys()) print(keys)
output
['name', 'age', 'city']
Retrieving Values
Similarly, the values() method returns a view object containing all the values of a dictionary. We can convert this view object into a list as well. Let’s see an example:
my_dict = {'name': 'Sandesh', 'age': 24, 'city': 'Delhi'} values = list(my_dict.values()) print(values)
output
['Sandesh', 24, 'New Delhi']
Conclusion
In Python, a common task when working with data is to retrieve keys and values from dictionaries. In this article, we discussed different methods to retrieve keys and values, including loops and dictionary methods. Now, you have a solid understanding of how to retrieve dictionary keys and values in Python. By using loops and dictionary methods like keys() and values(), you can efficiently access the desired information from your dictionaries.
Remember, dictionaries in Python are versatile data structures that can hold a wide range of data types as values. Whether you’re working with user information, inventory data, or any other type of information that requires key-value storage, dictionaries provide a flexible solution.
Apart from the methods which we have discussed in this article, Python also offers multiple other functionalities to manipulate dictionaries. You can try and experiment with other methods like items(), which allows you to retrieve both the key-value pairs simultaneously, or use conditional statements to filter specific keys or values based on certain criteria.
Python’s extensive documentation is an excellent resource to further expand your knowledge on dictionary operations and explore additional functionalities.
Popular Posts
- A Comprehensive Guide on Hyperparameter Tuning
- Python for Machine Learning: A Beginner’s Guide
- Mastering Image Contrast: A Step-by-Step Guide to Enhancing Image
- Histogram Equalization: Enhancing Image Quality and Contrast