11 Tips to Instantly Improve Your Python Code
Naveen
- 0
Python is a powerful programming language known for its simplicity and readability. In this article, we will explore 11 tips that can instantly improve your Python code. These tips include best practices that make your code cleaner and more pythonic.
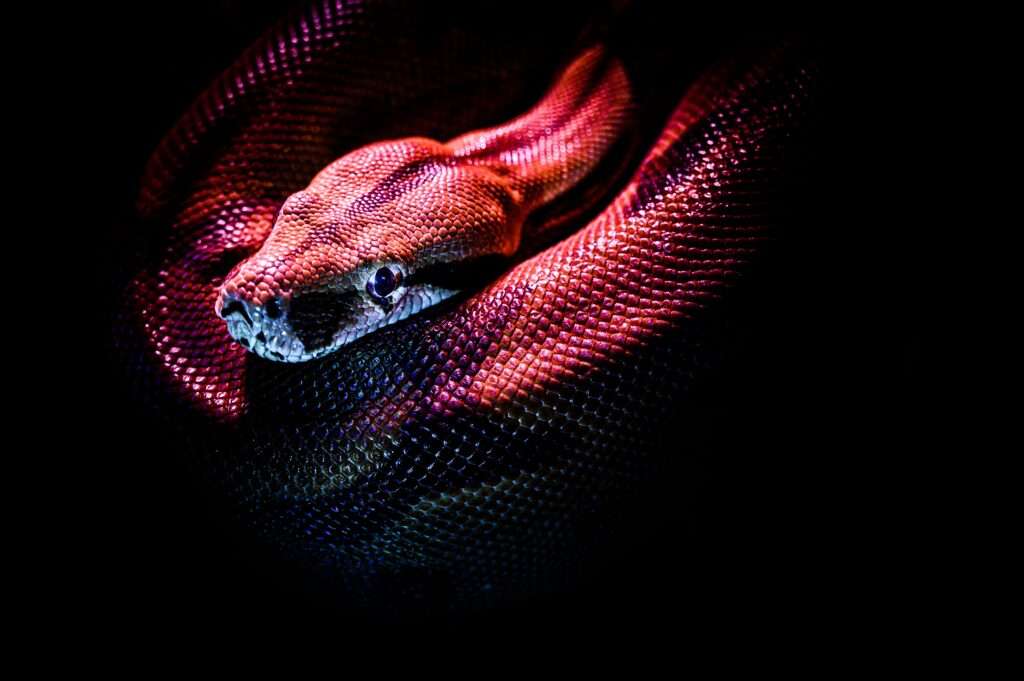
Tip 1: Iterate with `enumerate` instead of `range(len())`
When you need to iterate over a list and track both the index and the current item, most people use the `range(len())` syntax. However, a better alternative is to use the built-in `enumerate` function.
my_list = ['apple', 'banana', 'cherry'] # Using range(len()) for i in range(len(my_list)): print(i, my_list[i]) # Using enumerate for i, item in enumerate(my_list): print(i, item)
By using `enumerate`, you can directly access the index and the item as a tuple, making your code cleaner and more readable.
Tip 2: Use List Comprehension instead of raw for loops
List comprehension is a powerful feature in Python that allows you to create lists in a more concise and efficient way.
# Creating a list with squared numbers using a for loop squared_numbers = [] for i in range(10): squared_numbers.append(i**2) # Using list comprehension squared_numbers = [i**2 for i in range(10)]
As you can see, list comprehension reduces the code to a single line, making it easier to read and understand. It can also include if statements for more complex filtering.
Tip 3: Sort complex iterables with the built-in `sorted` method
Sorting iterables like lists, tuples, or dictionaries can be a tedious task. However, Python provides a built-in `sorted` function that simplifies the process.
my_list = [4, 2, 1, 3] # Sorting a list in ascending order sorted_list = sorted(my_list) print(sorted_list) # Output: [1, 2, 3, 4] # Sorting a list in descending order sorted_list = sorted(my_list, reverse=True) print(sorted_list) # Output: [4, 3, 2, 1]
The `sorted` function works on any iterable and returns a new sorted list. You can also specify the sorting key using the `key` argument.
Tip 4: Store unique values with sets
If you have a list with multiple values and need to have only unique values, you can use sets. Sets are an unordered collection data type that automatically removes duplicate elements.
my_list = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4] # Converting a list to a set unique_set = set(my_list) print(unique_set) # Output: {1, 2, 3, 4}
By converting your list to a set, you can easily remove duplicate elements and perform set operations like intersections and differences.
Tip 5: Save memory with generators
While list comprehension is a powerful tool, it may not always be the best choice, especially when dealing with large data. In such cases, generators can be more memory-efficient.
# Calculating the sum of a large list using a list my_list = [i for i in range(10000)] sum_list = sum(my_list) # Calculating the sum of a large list using a generator my_generator = (i for i in range(10000)) sum_generator = sum(my_generator) print(sum_list) # Output: 49995000
Generators produce elements lazily, meaning they only generate one item at a time when requested. This can save a significant amount of memory when working with large datasets.
Tip 6: Define default values in dictionaries with `get()` and `setdefault()`
When working with dictionaries, you may encounter situations where a key may not exist. Instead of raising a `KeyError`, you can use the `get()` method to return a default value.
my_dict = {'item': 'apple', 'price': 1.99} # Getting the count of items with a default value count = my_dict.get('count', 0) print(count) # Output: 0 # Updating the dictionary with a default value count = my_dict.setdefault('count', 0) print(count) # Output: 0
The `get()` method returns the value for the specified key, or a default value if the key is not found. Similarly, the `setdefault()` method returns the value for the specified key, and if the key is not found, it adds the key-value pair to the dictionary.
Tip 7: Count hashable objects with `collections.Counter`
If you need to count the number of elements in a list, the `collections` module provides a handy tool called `Counter`.
from collections import Counter my_list = ['apple', 'banana', 'apple', 'cherry'] # Counting the number of elements in a list counter = Counter(my_list) print(counter) # Output: Counter({'apple': 2, 'banana': 1, 'cherry': 1}) # Getting the count for a specific item count = counter['apple'] print(count) # Output: 2 # Getting the most common items most_common = counter.most_common(2) print(most_common) # Output: [('apple', 2), ('banana', 1)]
The `Counter` object allows you to count the occurrences of each element in a list. It also provides useful methods like `most_common()` to retrieve the most common items.
Tip 8: Format strings with F-strings
Since Python 3.6, a new string formatting method called F-strings has been introduced. F-strings provide a concise and efficient way to format strings.
name = "Rohit" age = 25 # Formatting strings with F-strings message = f"My name is {name} and I am {age} years old." print(message) # Output: "My name is Rohit and I am 25 years old."
By prefixing the string with an `f`, you can include variables directly inside curly braces. You can even write expressions inside the curly braces, which will be evaluated at runtime.
Tip 9: Concatenate strings with `join()`
When you have a list of strings and want to combine them into a single string, you can use the `join()` method. This method is more efficient than manually concatenating strings.
my_list = ['Hello', 'World', '!'] # Concatenating strings with join() result = ' '.join(my_list) print(result) # Output: "Hello World !"
By using the `join()` method, you can specify the separator between each word. In this example, we used a space as the separator.
Tip 10: Merge dictionaries with the double asterisk syntax
If you have two dictionaries and want to merge them into one, you can use the double asterisk syntax.
dict1 = {'name': 'John', 'age': 25} dict2 = {'city': 'New York'} # Merging dictionaries with the double asterisk syntax merged_dict = {**dict1, **dict2} print(merged_dict) # Output: {'name': 'John', 'age': 25, 'city': 'New York'}
By using curly braces and the double asterisk syntax, you can easily merge dictionaries into a single dictionary.
Tip 11: Simplify if statements with `if X in list`
When you need to check if a variable matches any item in a list, you can simplify the if statement using the `in` operator.
main_colors = ['red', 'green', 'blue'] color = 'red' # Simplifying if statements with `if X in list` if color in main_colors: print("Color found!") else: print("Color not found!")
By using `if X in list`, you can check if the variable matches any item in the list without writing separate if statements for each item.
Conclusion
In this blog post, we explored 11 tips that can instantly improve your Python code. By following these tips, you can write cleaner, more efficient, and more pythonic code. I hope you found these tips helpful and learned something new. If you have any other good tips, please let me know in the comments.
I hope this article has been helpful to you, thanks for reading this article.
Popular Posts
- From Zero to Hero: The Ultimate PyTorch Tutorial for Machine Learning Enthusiasts
- Day 3: Deep Learning vs. Machine Learning: Key Differences Explained
- Retrieving Dictionary Keys and Values in Python
- Day 2: 14 Types of Neural Networks and their Applications