Advanced String Manipulation with Pandas
Naveen
- 0
Pandas is a popular Python library for data analysis that provides powerful techniques for data manipulation, cleaning, and exploration. One of the most useful features of Pandas is its ability to handle string data. In this article, we will explore advanced string manipulation techniques using Pandas.
1 – Splitting and Extracting Strings
One of the most common tasks when working with string data is splitting or extracting parts of a string. Pandas provides several functions for this, including str.split() and str.extract(). The str.split() function splits a string into a list of substrings based on a delimiter. For example:
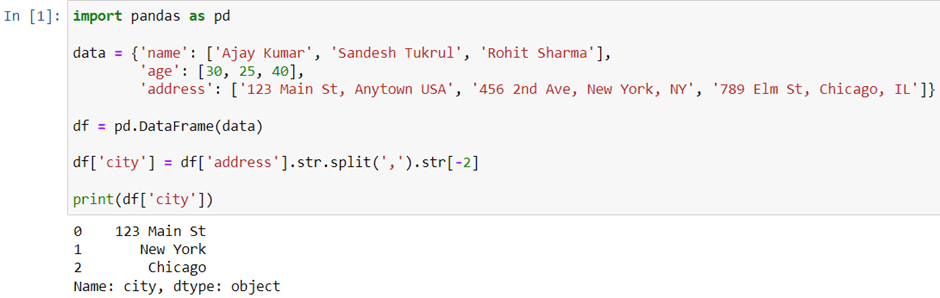
This code uses the str.split() function to split the address column into a list of substrings based on the comma delimiter. We then use the str[-2] index to extract the second-to-last substring, which is the city.
2 – Replacing and Cleaning Strings
Another common task when working with string data is replacing or cleaning strings. Pandas provides several functions for this, including str.replace() and str.strip(). The str.replace() function replaces occurrences of a substring with another substring. For example:

The str.strip() function removes leading and trailing whitespace from a string. For example:
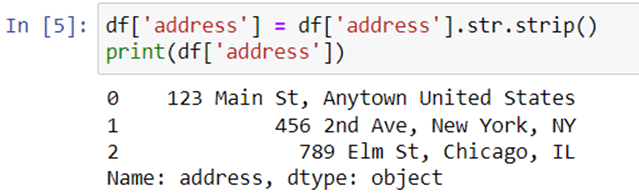
3 – Concatenating Strings
Sometimes it is necessary to concatenate strings together to create a new column. Pandas provides the str.cat() function for this. For example:
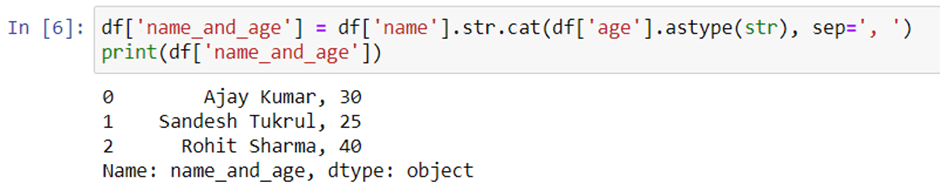
This code concatenates the name and age columns together with a comma and space separator to create a new name_and_age column.
4 – Splitting Strings
We can split strings in a DataFrame into multiple columns using the str.split() method. For instance, consider a DataFrame that contains a column with full names. We may want to split this column into separate columns for first name and last name. We can use the following code to split the names:
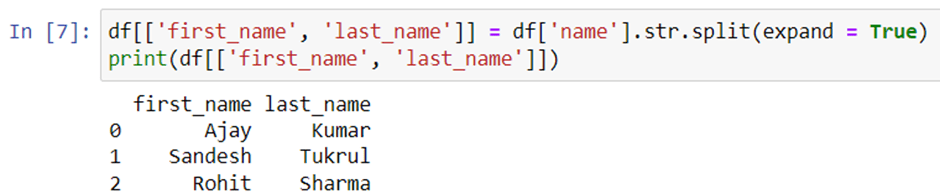
5 – Fuzzy Matching
Fuzzy matching is a technique for finding approximate matches between strings. This can be useful when dealing with typos, misspellings, or variations in data.
Pandas provides the fuzzywuzzy library for fuzzy matching, which can be installed using pip. Here’s an example of how to use fuzzywuzzy to match names in a DataFrame:
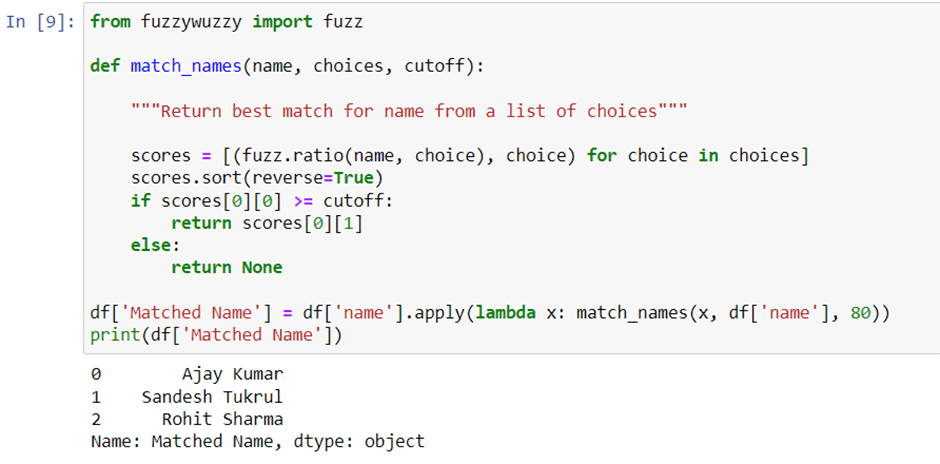
Conclusion
In this article, we have explored advanced string manipulation techniques using Pandas. We have covered splitting and extracting strings, replacing and cleaning strings, and concatenating strings. These techniques are useful for cleaning and transforming string data in a variety of data analysis tasks. With the power of Pandas, we can efficiently perform complex string operations on large datasets.