OOPs in Python
Naveen
- 0
Object-oriented programming (OOP) is a programming paradigm based on the concept of objects which contains data or attributes and code or methods.
Major oops concepts include Class, Object, Inheritance, Polymorphism, Data Abstraction, Encapsulation.
Class
Class is the user defined data type and it is defined using class keyword. It is a collection of similar type of objects.
When the class is created, memory is not allocated to it but when its instance is created memory is allocated.
Object
Object is an instance of class. Once class is defined you can create any number of objects. Objects contains the data and function to manipulate the data
Object can have attributes such as name or age of student and behavior such as whether the student is completing the given tasks or not.
Syntax;
Object = class_name()
Example: Creating class and object

Here __init__() is the initializer method which gets called as soon as the object is created. This is similar to constructors in C++ and java.
Each method definition in class should have an extra first parameter that is self. We do not require to provide any value for self parameter while calling the method. Self is the must have parameter in method even if that method does not take any other parameters.
Method
Methods are functions defined inside a class that enable operations on instance objects and/or.
- Instance methods
Inside a class, instance method differ from other methods through their first argument which must be the reference to the instance. By convention, the word self, which is not a Python keyword, is used in Python to introduce the reference to an instance itself.
The class person shows now more functionality with the added method, but without an instance, a call to the method greet will always fail and it is the case by all instance methods.
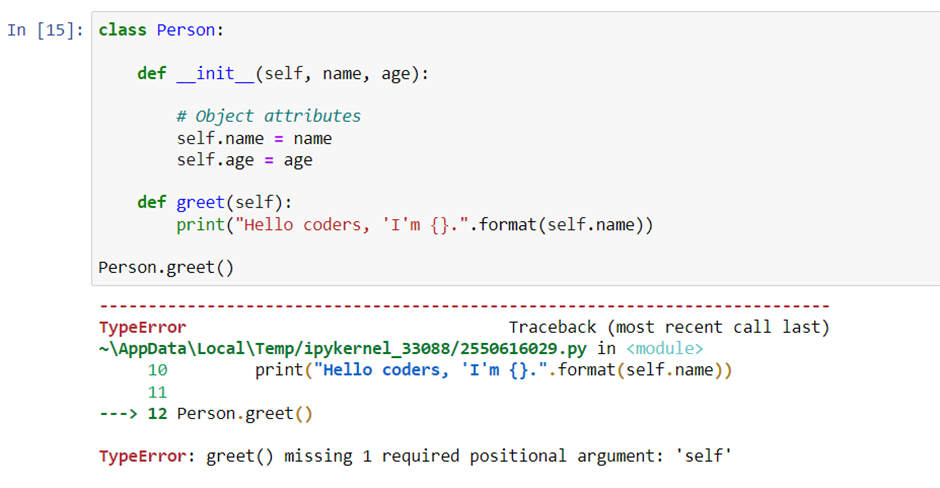
If you call this method without creating an instance you would get above error.
As we can see, the error indicates that the method is missing the argument self because the call was executed without an object instance:
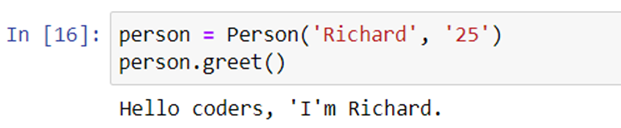
- Class methods
A class method is identified inside a class through the decorator @classmethod preceding the method definition. In addition, a class method has an implicit first argument standing for reference to the calling class. The world cls, which is also not a Python keyword, is used by convention to represent the argument. Class method can access and modify the state of a class but cannot change the state of class instances individually however the changes made to the state of a class are directly applied to all instances.
The example below shows the definition of the class method patient for the initialization of objects of the class Person with the additional property female or male:

Using our above-created object person we can also access the static method and create a new instance of Person:

- Static methods
A static method in Python is similar to a class level method, the only difference being that it is bound to a class rather than an object for that class You’re able to call static methods without knowing the class or an instance of that class. Static methods cannot modify the state of an object because they are not bound to it.
Creating python static methods
Python Static methods can be created in two ways:
Using staticmethod()
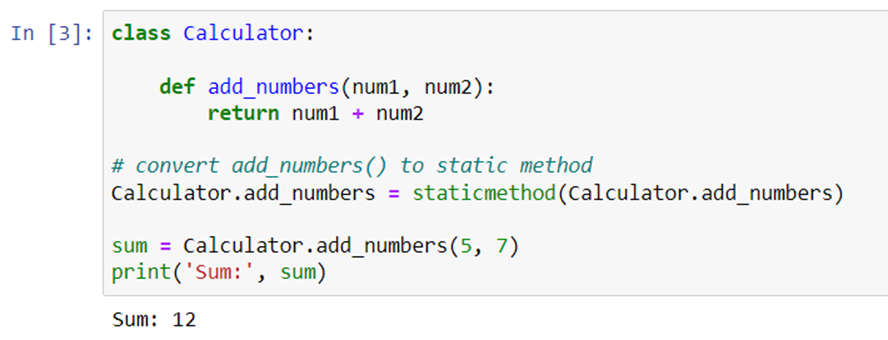
Using @staticmethod
This is a more subtle way of creating a static method as we do not have to rely on a statement definition of the method being a class method and emphasizing as such in each place it should be made static. We can use this annotation in the following code snippet:
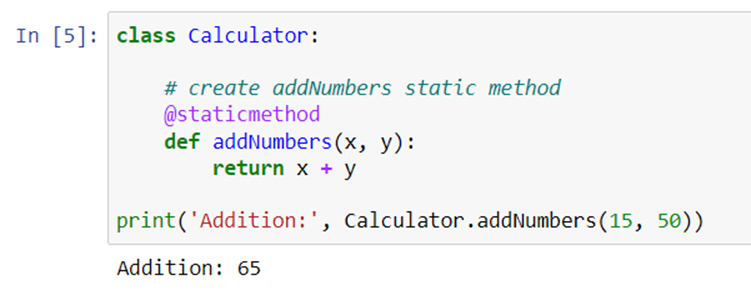
inheritance
It is the process in which object of one class can acquire the properties of object of another class.
Inheritance provides the reusability which means that we can add more features to an existing class without modifying it.
We can create the new class from the existing class. The newly created class is known as base class or parent class.
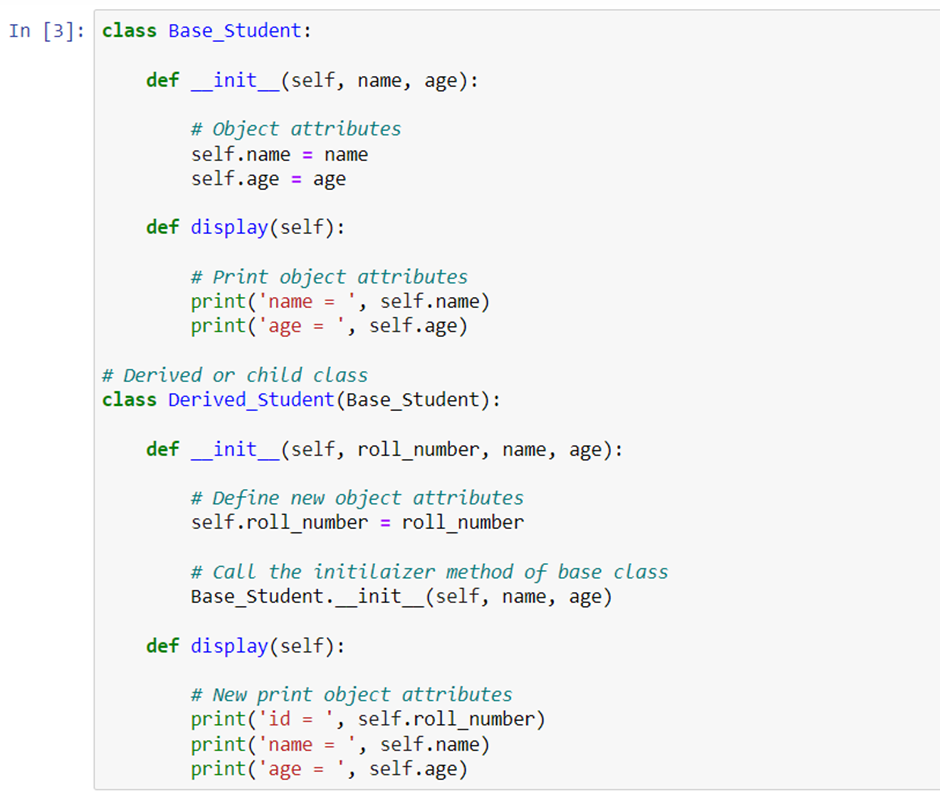

Polymorphism
Polymorphism is an ability to take more than one tasks. It is achieved by using function overloading in which a function can have same name but different functionalities.
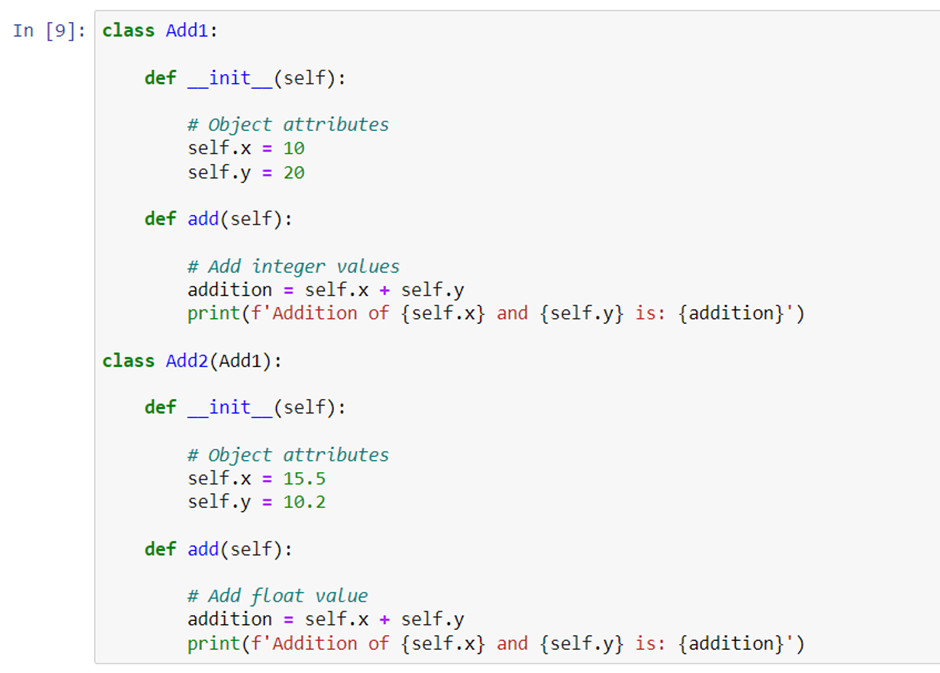
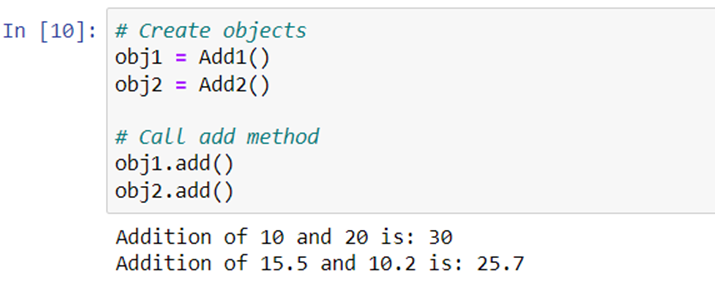
Encapsulation
The binding of data and function into a single unit (class) is known as encapsulation.
The data is not accessible to outside of the class, it is accessible to only those functions which are defined in the same class.
In this data defined in the class is hidden from outside, hence it is also known as data-hiding or information hiding.
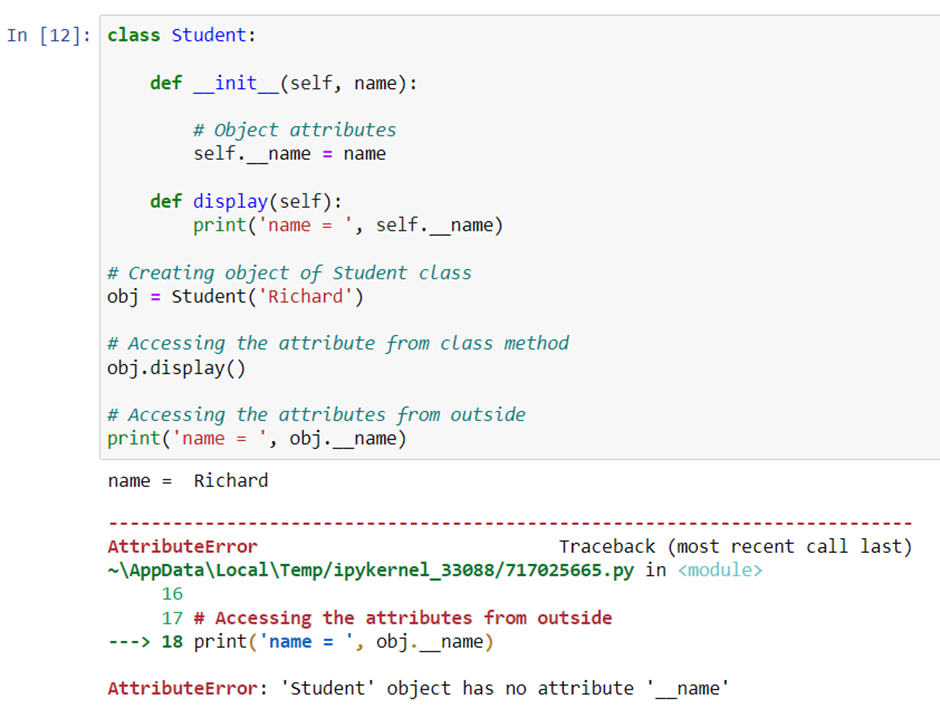
Here double underscore in the prefix of name attribute denotes that it is a private member. So it can be accessed from display() method but not from outside, so it will raise AttributeError for obj.__name.