Abstraction in Python
Naveen
- 0
Abstract Class
- Abstract classes are classes that contain one or more abstract methods.
- An abstract method is declared, but contains no implementation.
- Abstract classes cannot be instantiated, and require subclasses to provide implementations for the abstract methods.
- Subclasses of an abstract class in Python are not required to implement abstract methods of the parent class.
- Python provides the abc module to use the abstraction in the Python program.
Why Abstraction is Important?
In Python, a person usually abstracts data/classes to hide the irrelevant information. This helps to reduce complexity and increase application efficiency.
Abstract Base Classes
An abstract class is the first part of a development interface that can be used to apply common features and behavior to several related subclasses. For example, plugins would implement this part of the interface so that you can use them as needed with your code. This type of class is also beneficial when you are working with a large set of code because it reduces overlap and interdependency.
The Working of Abstract Classes
Python won’t automatically provide you with the ABC classes. You’ll need to import the abc module before defining your class. The ABC software works by decorating the methods of a base class as abstract and registering concrete classes as the implementations of that base class. We use the @abstractmethod decorator to define an abstract method or if we don’t provide the definition to the method, it automatically becomes the abstract method.
As a property, abstract classes can have any number of abstract methods coexisting with any number of other methods. For example we can see below.
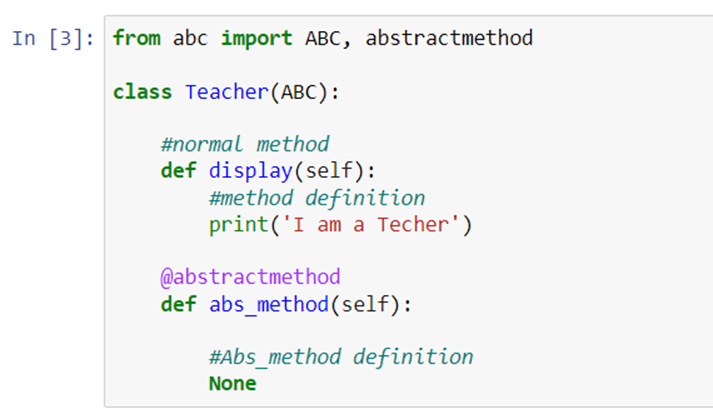
Here, display()
is normal method whereas abs_method()
is an abstract method implementing @abstractmethod
from the abc module.
Python Abstraction Example
Now that we know about abstract classes and methods, let’s take a look at an example which explains Abstraction in Python.
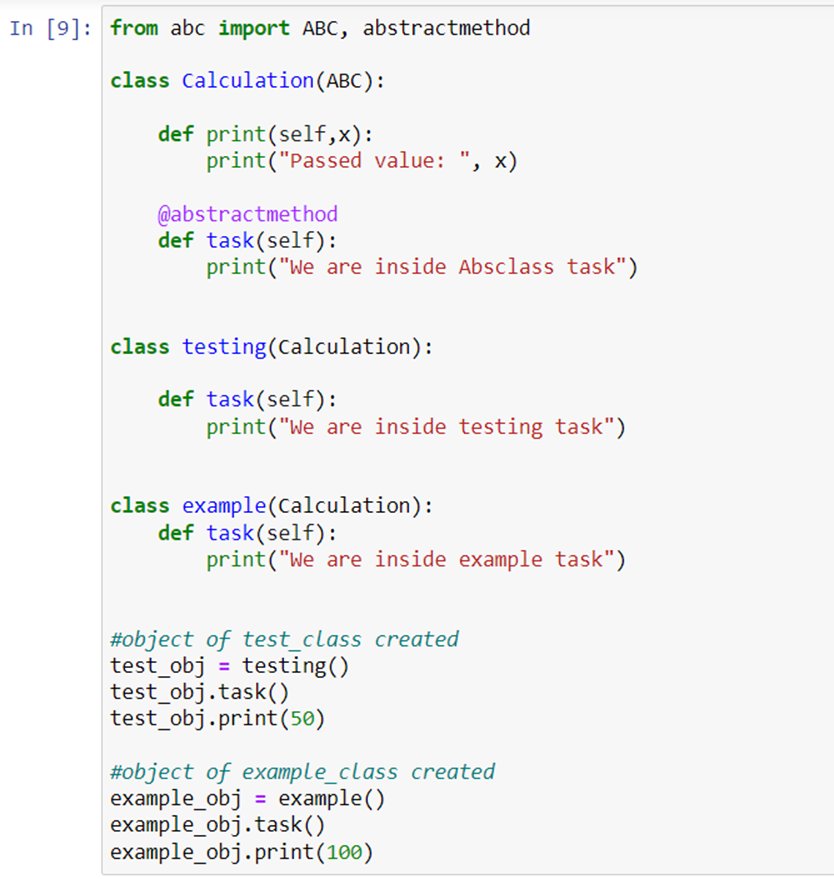
Here,
Calculation is the abstract class that inherits from the ABC class from the abc module. It contains an abstract method task() and a print() method which are visible by the user. Two other classes inheriting from this abstract class are testing and example. Both of them have their own task() method (extension of the abstract method). After the user creates objects from both the test_class and example_class classes and invoke the task() method for both of them, the hidden definitions for task() methods inside both the classes come into play. These definitions are hidden from the user. The abstract method task() from the abstract class Calculation is actually never invoked.
But when the print() method is called for both the example_obj and example_obj, the Calculation’s print() method is invoked since it is not an abstract method.