Python Basics
Naveen
- 0
Let’s quickly explore the first tools and basic syntax rules for a beginner to start using Python. But before that, know that when needed, you can find documentation on the Python.org site and many online resources, including sites like StackOverflow.
Printing things
We use the print() function to print stuff in Python. This is essential to handle and provide output for our programs, so let’s start learning how to use it right away.


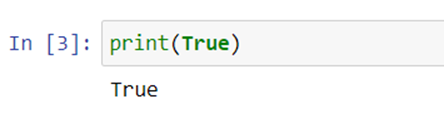

Variables
Since we build a program using Python language, we need a way to reference values that our program manipulates. That is the purpose of variables. We use a variable to reference a value. And later in our program, we call a given variable whenever we need the value it references.


Now that we know how to define variables, let’s see another important element of Python:
Code Indentation
Python does not use {} for code blocks, but text indentation. We use a number of space characters, in a consistent way, to indent each block of code. 4 spaces indentation is the recommended practice, though using 2 spaces would also work.
To visualize things, here is an example of pseudo-code:
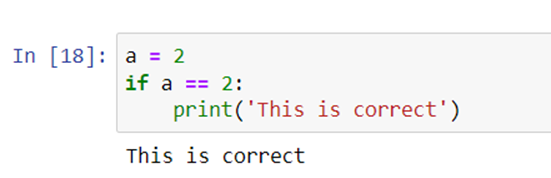
This is important to understand that indentation is part of the language. If the bad indentation is found by the interpreter, it will throw a syntax error. The example below shows what happens with code that contains bad indentation:

The version without error would as follow:

Indentation in Python can be confusing at first, but you get used to it once you practice writing Python code every day.
Simple data types
Any program you will write manipulate data in some way. Let’s look at the first data types which one may use to do something.
Numeric data
In this category, we have number of specific types such as integer and float, which you can also find in other similar programming languages.
- Int type: the int is the type used to create and manipulate integer numbers such as 0, 1, 100, 355, 1200, and any more. The following code example help you discover and manipulate int objects:


- Float type: The float is the type used to create and manipulate floating point numbers such as 1.5, 3.5, 5.7. 2.8, and many more. The following code examples help you discover and manipulate float objects:


- Strings – The string is the type we use to handle text data by default. A string is a sequence of character. Being a sequence type makes it practical to work with strings since we can access each member of the sequence and also do many operations available for sequence.
There are several ways to define a variable containing a string, as we can see in the following examples:

As we said earlier, one important thing to understand is that strings are sequences. So, you can access a string’s members using its index. We will see later in more detail the characteristics and techniques related to sequences but here are quick examples.
You can access characters in the string like the following example shows:

You can also calculate the length of a string, using a python built-in function called len(), as shown below:

- Booleans : Like in other programming languages, Booleans are the two values True or False we can use for condition expressions:

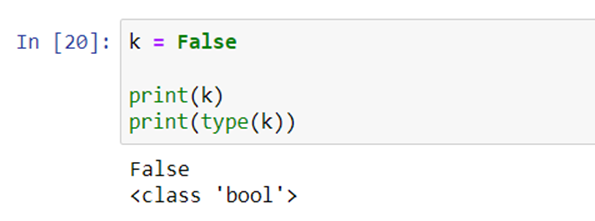