Enhancing Image Visibility with Adaptive Thresholding
Naveen
- 0
In this article we will be working on Enhancing Image Visibility with Adaptive Thresholding. You must have come across images that appear too dark or lack the necessary contrast to make out important details? Whether it’s a poorly lit photograph or a scanned document, such images can be challenging to interpret. Fortunately, there’s a powerful technique called adaptive thresholding that can help us overcome this problem and bring out the hidden details in our visuals. In this article, we will using adaptive thresholding and its application to enhance image visibility.

Understanding Adaptive Thresholding
Adaptive thresholding is an image processing technique used to separate the foreground from the background by dynamically adjusting the threshold value based on the local characteristics of the image. Unlike global thresholding, which uses a fixed threshold for the entire image, adaptive thresholding adapts the threshold value on a pixel-by-pixel basis. This allows us to handle variations in lighting conditions and improve the visibility of objects in the image.
Code Implementation:
To see the example of adaptive thresholding, let’s take a look at a code snippet that applies this technique to an image:
import cv2 import numpy as np import matplotlib.pyplot as plt # Read the original image img_original = cv2.imread('dark.png') img = cv2.cvtColor(img_original, cv2.COLOR_BGR2GRAY) # Apply adaptive thresholding adaptive_thresh = cv2.adaptiveThreshold(img, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY, 201, 3) # Create a figure with two subplots side by side fig, axs = plt.subplots(1, 2, figsize=(12, 6)) # Display the original image in the first subplot axs[0].imshow(cv2.cvtColor(img_original, cv2.COLOR_BGR2RGB)) axs[0].set_title('Original Image') # Display the adaptive thresholded image in the second subplot axs[1].imshow(adaptive_thresh, cmap='gray') axs[1].set_title('Adaptive Threshold') # Adjust spacing between subplots plt.tight_layout() # Show the figure plt.show()
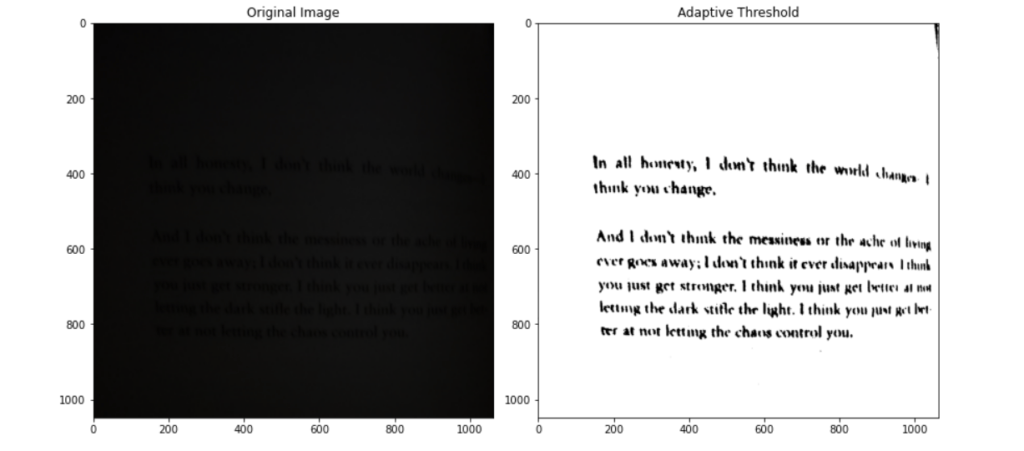
In this code, we start by loading the original image and converting it to grayscale for simplicity. We then apply adaptive thresholding using the cv2.adaptiveThreshold
function with parameters such as the maximum pixel value (255), the adaptive thresholding method (cv2.ADAPTIVE_THRESH_GAUSSIAN_C
), the thresholding type (cv2.THRESH_BINARY
), the block size (201), and the constant subtracted from the mean (3). These values can be adjusted depending on the specific image and desired results.
Next, we utilize the matplotlib
library to create a figure with two subplots side by side. The first subplot displays the original image, while the second subplot showcases the image after adaptive thresholding. By comparing these images, we can witness the substantial improvement in visibility and the enhanced differentiation between foreground and background.
Additional Applications and Benefits of Adaptive Thresholding
Adaptive thresholding has a wide range of applications beyond enhancing image visibility. Let’s see few of its benefits:
- Text Extraction: Adaptive thresholding can be used to extract text from images, making it valuable in OCR (Optical Character Recognition) applications. By thresholding the image to highlight the text, it becomes easier to extract and recognize characters accurately.
- Document Enhancement: When dealing with scanned documents that may have inconsistent lighting or faded text, adaptive thresholding can help enhance the document’s legibility. It improves the contrast between the text and background, making it easier to read and process the information.
- Object Detection: Adaptive thresholding can be an essential step in object detection algorithms. By segmenting the image into foreground and background, it helps isolate objects of interest and removes unnecessary details, simplifying subsequent analysis and recognition tasks.
- Image Preprocessing: Adaptive thresholding can be employed as a preprocessing step before applying other image processing techniques. By emphasizing important features and reducing noise, it can enhance the effectiveness of subsequent operations like edge detection or contour extraction.
Conclusion
Adaptive thresholding is a valuable technique in image processing that helps us to overcome challenges posed by poorly lit or low-contrast images. By dynamically adjusting the threshold value based on local image characteristics, adaptive thresholding enhances visibility and brings out important details that might otherwise remain hidden. Whether it’s enhancing photographs, improving scanned documents, or assisting in computer vision tasks, this technique has diverse applications.
So, the next time you encounter an image that appears too dark or lacks clarity, remember the adaptive thresholding technique. With just a few lines of code, you can improve the visibility of your image.
If you found this article helpful and insightful, I would greatly appreciate your support. You can show your appreciation by clicking on the button below. Thank you for taking the time to read this article.
Popular Posts
- From Zero to Hero: The Ultimate PyTorch Tutorial for Machine Learning Enthusiasts
- Day 3: Deep Learning vs. Machine Learning: Key Differences Explained
- Retrieving Dictionary Keys and Values in Python
- Day 2: 14 Types of Neural Networks and their Applications