How to Enhance Images Using Python
Naveen
- 0
Image enhancement is one of the important steps in number of applications, including computer vision and image processing. It will help you to improve the quality of an image by adjusting its brightness, contrast and sharpness. Python, a versatile programming language, that provides a number of libraries to perform image enhancement tasks. In this article, we will be implementing it using libraries like OpenCV and Pillow.
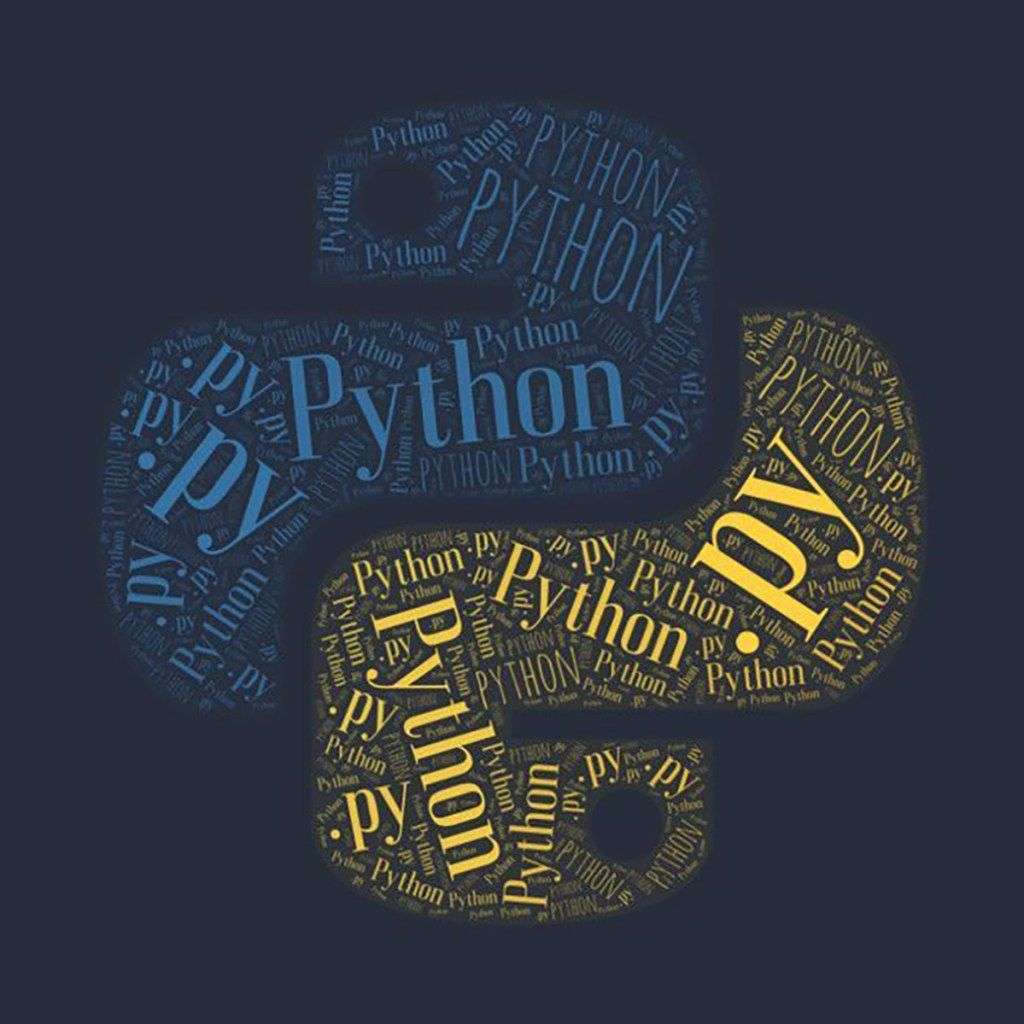
1 – Getting Started
So, before we start implementing the code, make sure to have Python installed on your system. You can download the latest version of Python from the official website: https://www.python.org/downloads/
Next, install the required libraries using pip:
pip install opencv-python pip install pillow
2 – Enhancing Images with OpenCV
OpenCV is a powerful library for computer vision and image processing. It provides various functions to manipulate and enhance images. Let’s implement few of these functions to improve image quality.
a – Adjusting Brightness and Contrast
To adjust the brightness and contrast of an image, we can use the cv2.addWeighted() function. This function takes the below parameters:
- src1: The first source image.
- alpha: The weight of the first source image.
- src2: The second source image.
- beta: The weight of the second source image.
- gamma: A scalar added to each sum
Let’s see an example of how to use this function:
import cv2 import numpy as np import matplotlib.pyplot as plt def adjust_brightness_contrast(image, alpha, beta): return cv2.addWeighted(image, alpha, image, 0, beta) image = cv2.imread('dog.jpg') enhanced_image = adjust_brightness_contrast(image, 1.2, 30) original_and_enhanced_image = np.hstack((image, enhanced_image)) plt.figure(figsize = [30, 30]) plt.axis('off') plt.imshow(original_and_enhanced_image [:,:,::-1])
After executing above code, you will get the following output:
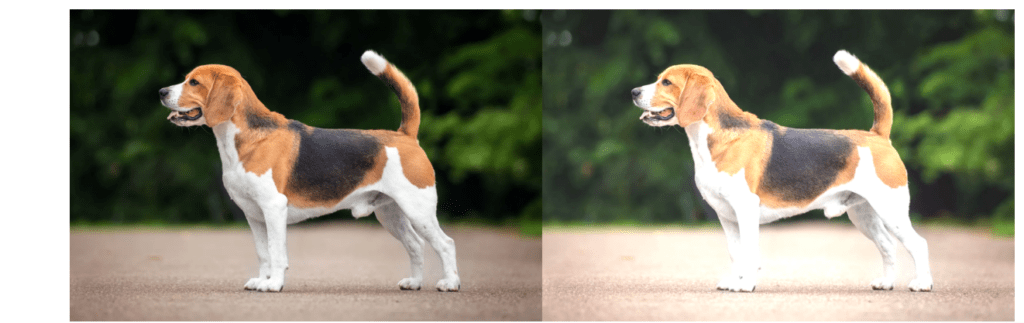
In this example, we increase the brightness by 30 and the contrast by 1.2.
b. Sharpening Images
If we want to sharpen an image, we can use the cv2.filter2D() function, which applies a linear filter to the image. We will use a sharpening kernel to enhance the image details.
import cv2 import numpy as np import matplotlib.pyplot as plt def sharpen_image(image): kernel = np.array([[-1, -1, -1], [-1, 9, -1], [-1, -1, -1]]) return cv2.filter2D(image, -1, kernel) image = cv2.imread('dog.jpg') sharpened_image = sharpen_image(image) original_and_sharpened_image = np.hstack((image, sharpened_image)) plt.figure(figsize = [30, 30]) plt.axis('off') plt.imshow(original_and_sharpened_image[:,:,::-1])
After executing above code, you will get following output:
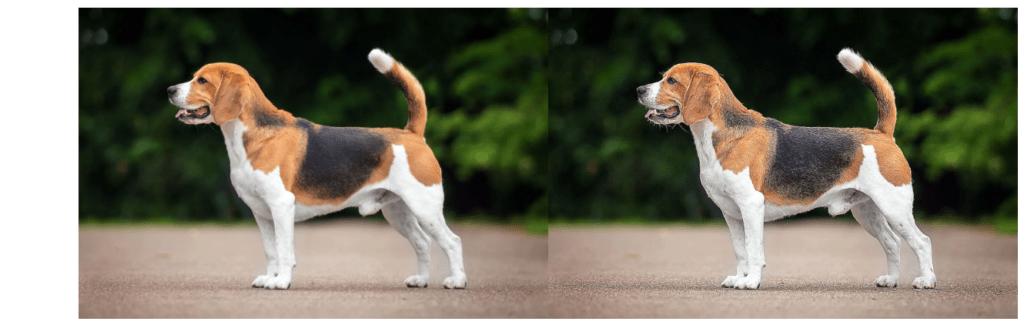
3 – Enhancing Image with Pillow
Pillow (Python Imaging Library) is another popular library for image processing in Python. It provides a user-friendly interface to work with images and offers various functions to enhance them.
a. Adjusting Brightness
If we want to adjust the brightness of our image, we can use the ImageEnhance.Brightness class from the PIL.ImageEnhance module. This class takes an image as input and provides an enhance() method to adjust the brightness.
from PIL import Image, ImageEnhance import numpy as np import matplotlib.pyplot as plt def adjust_brightness(image, factor): enhancer = ImageEnhance.Brightness(image) return enhancer.enhance(factor) image = Image.open('dog.jpg') enhanced_image = adjust_brightness(image, 1.5) original_and_enhanced_image = np.hstack((image, enhanced_image)) plt.figure(figsize = [30, 30]) plt.axis('off') plt.imshow(original_and_enhanced_image, cmap = 'gray')
In this example, we increase the brightness by a factor of 1.5.
After executing above code, you will get following output:
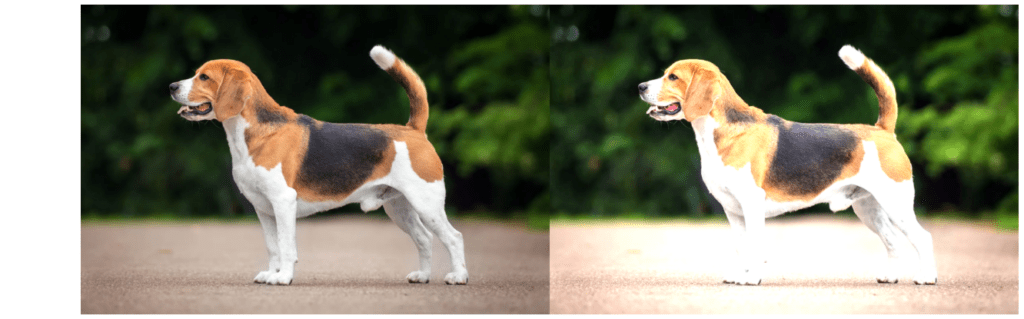
b. Adjusting Contrast
To adjust the contrast of an image, we can use the ImageEnhance.Contrast class from the PIL.ImageEnhance module. This class takes an image as input and provides an enhance() method to adjust the contrast.
from PIL import Image, ImageEnhance import numpy as np import matplotlib.pyplot as plt def adjust_contrast(image, factor): enhancer = ImageEnhance.Contrast(image) return enhancer.enhance(factor) image = Image.open('dog.jpg') enhanced_contrast = adjust_contrast(image, 1.5) original_and_enhanced_contrast = np.hstack((image, enhanced_contrast)) plt.figure(figsize = [30, 30]) plt.axis('off') plt.imshow(original_and_enhanced_contrast, cmap = 'gray')
In this example, we increase the contrast by a factor of 1.5.
After executing above code, you will get following output:

Conclusion
In this article, we discussed how to enhance images using Python with the help of OpenCV and Pillow libraries. We covered various techniques, including adjusting brightness, contrast, and sharpening images. These libraries offer a wide range of functions to manipulate and enhance images, making Python a powerful language for image processing tasks.
I hope you liked this article, if you have any question let me know.
Popular Posts
- A Comprehensive Guide on Hyperparameter Tuning
- Python for Machine Learning: A Beginner’s Guide
- Mastering Image Contrast: A Step-by-Step Guide to Enhancing Image
- Histogram Equalization: Enhancing Image Quality and Contrast