Operations in Python
Naveen
- 0
There are many type of different operations using operators in the language:
- identity using “is”
- Variable assignment using “=”
- Comparison operations
- Arithmetic operations
- operations on sequences
- Logical operations
Identity
As we manipulate values (using variable), two values can have the same identity. This can be tested using special built-in function: id().
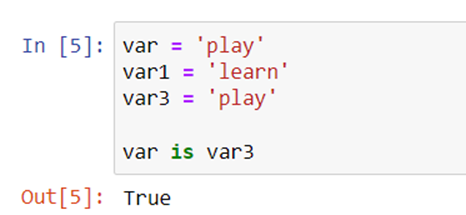
We use is as the identity comparison operator, and the result here shows that var and the var3 have the save identity. Two variable referencing the same value, the play string.
We can also show the visually:
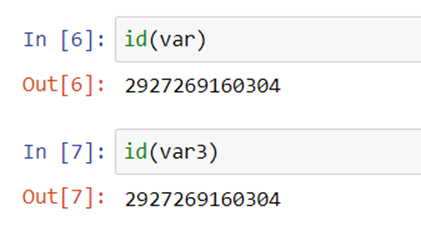
And, for the same reasons, var1 is not var3, as we can see below:
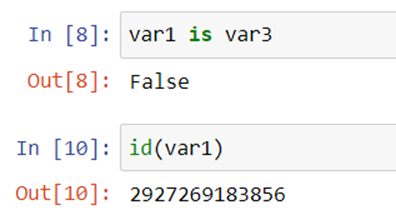
Variable assignment
We have already seen the operations when we introduced variables.
You assign a value or an expression (that will be evaluated) to a variable, using the = operator.
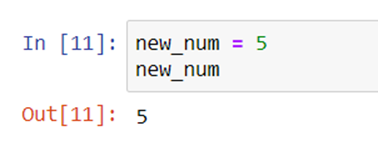
We can also assign the value of an existing variable to a new one.
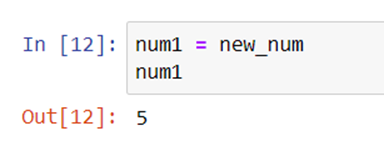
And we can evaluate an expression using existing variables and assign the result to a new variable:
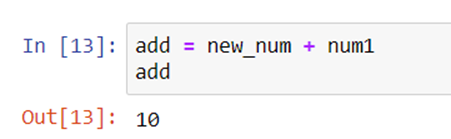
Comparison operations
We often need to compare two values. For that, we have equality and inequality test operators:
- == for equality: the evaluation of the comparison expression returns True if both values are equal.
- And >= for greater than: we get True if the value at the left is greater than (or greater than or equal to) the value at the right.
- < and <= for lesser than: we get True if the value at the left is lesser than (or lesser than or equal to) the one at the right.
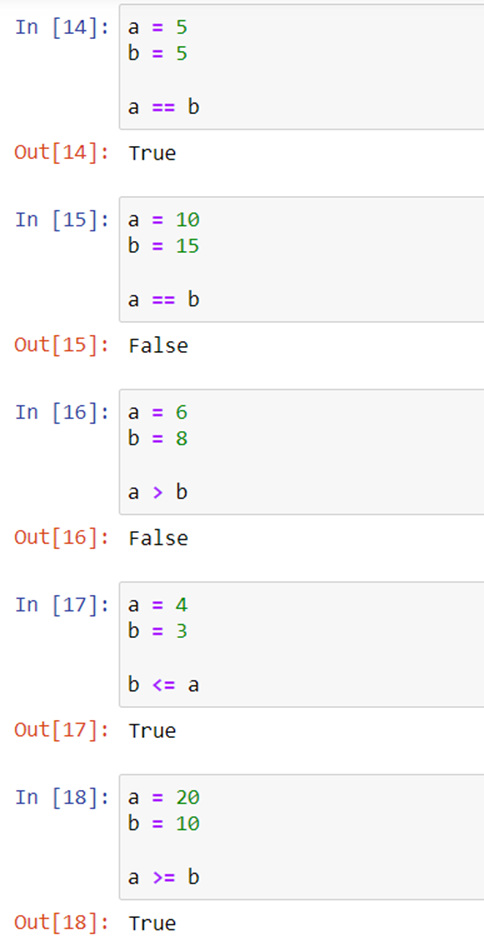
Arithmetic operations
Operators for arithmetic calculation such as addition are available in python like in other programming languages. They are very easy to use, and allow us to use the python interpreter as a calculator. Let’s see how this works:
- Addition: The first arithmetic operation one would think of is the addition. We use + to add numbers. For example:
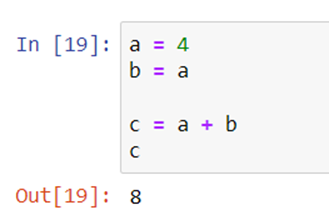
- Multiplication: we use * to multiply numbers. For example:
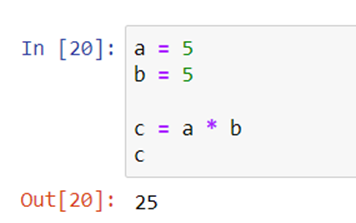
- Division: There are 3 operators for division: /, //, and %. The / operator returns the float number resulting from the division. Let’s see an example:
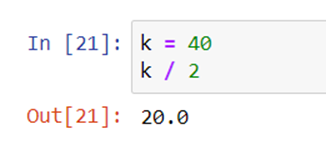
The // operator returns the integer resulting from the division. Let’s see an example:
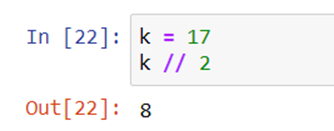
The % operator returns the remainder of the division. Let’s see an example:
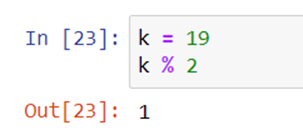
- Other arithmetic operations: we can use – for subtraction, as shown the following example:
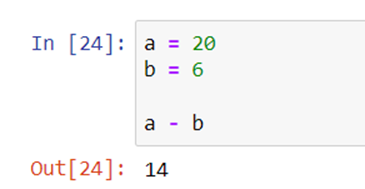
We can use ** to perform exponential (power). Let’s see an example:
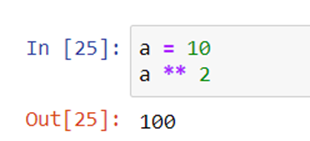
Operations on sequences
Some operations are possible with sequences such as string and lists. Let’s see which ones and how they work.
- String concatenation: we use + to concatenate strings. Here is a first example:
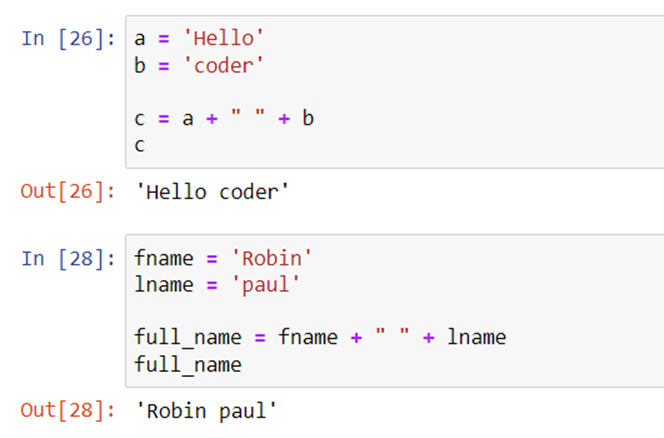
- String multiplication: we use * to multiply strings. Let’s see an example:

- List concatenation: we also use + to concatenate lists. Let’s see an example
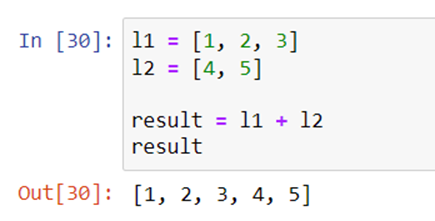